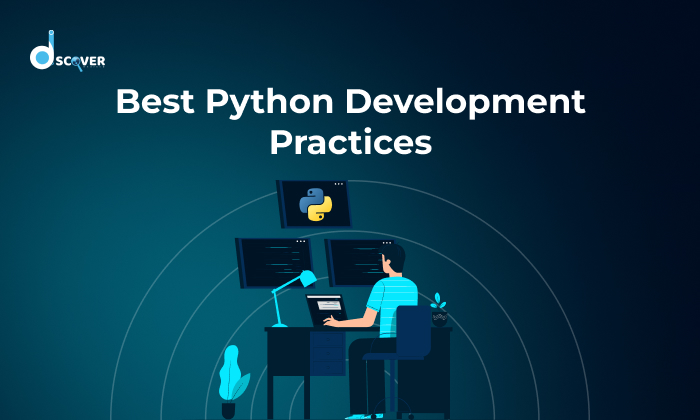
Python is a popular programming language used by developers worldwide. Whether you are a beginner or an experienced Python developer, following the best practices can help you write clean, efficient, and error-free code. In this guide, we will explore essential Python best practices that will improve your coding skills and help you build better applications.
Follow PEP 8 Guidelines
PEP 8 is the official style guide for writing Python code. It provides rules on how to format your code so that it looks clean and professional. Some key PEP 8 guidelines include:
- Use 4 spaces for indentation (instead of tabs).
- Keep line length up to 79 characters.
- Leave blank lines between different sections of code to improve readability.
Following PEP 8 makes your code easy to read and understand for you and others working on the same project.
Use Meaningful Variable Names
When writing code, always use clear and descriptive names for variables. This makes it easier to understand what the variable represents.
For example, instead of writing: a = 10
Write: user_count = 10
Using meaningful names helps in debugging and makes your code more readable.
Write Simple and Efficient Code
Python provides many features that allow you to write short and efficient code. For example, instead of using a loop to create a list of numbers, you can use a list comprehension to do it in one line.
Instead of:
- Creating an empty list and adding numbers one by one.
Do this:
- Create the list in a single line using list comprehension.
Writing short and optimized code makes your program run faster and looks cleaner.
Handle Errors Properly
Errors are a part of coding, but handling them properly prevents the program from crashing. Instead of letting the program stop when an error occurs, you can use exception handling to manage errors.
For example, if a user enters text instead of a number, your program should display a proper message instead of stopping.
Using proper error handling makes your program more reliable and user-friendly.
Test Your Code with Unit Tests
Testing your code is important to ensure it works correctly. Instead of manually checking if your code is working, you can write unit tests to automate the process.
Testing helps in:
- Finding mistakes early.
- Making sure new changes don’t break the existing code.
Regular testing improves the quality of your software and makes debugging easier.
Write Clear Comments and Documentation
Adding comments in your code helps others understand what your code does. Even if you come back to your project after months, good comments will remind you of its purpose.
For example, before a complex section of code, you can add a comment explaining what it does.
Besides comments, creating proper documentation for your project will help other developers understand how to use your code.
Use Version Control (Git)
When working on a project, using Git (a version control system) allows you to:
- Track changes in your code.
- Revert to an older version if needed.
- Work on different features without affecting the main code.
Version control is especially useful when working in teams, as it keeps the project organized and prevents accidental mistakes.
Keep Your Code Organized
A well-organized project is easier to maintain. Here are some tips to keep your code structured:
- Keep related functions and classes together.
- Use folders to separate different parts of your project.
- Name files properly so they are easy to identify.
An organized project saves time and effort in the long run.
Use Virtual Environments for Managing Dependencies
In Python development, you often use different libraries for different projects. A virtual environment helps you keep these dependencies separate so that one project’s settings do not affect another.
Using virtual environments makes it easier to manage your projects and avoid compatibility issues.
Follow the Zen of Python
Python follows a simple philosophy called the Zen of Python, which encourages developers to write code that is:
- Simple and clear.
- Easy to read and understand.
- Well-structured and efficient.
You can view these principles by running import this in a Python program. Keeping these ideas in mind will help you write better code.
Final Thoughts
By following these best practices, you can improve your coding skills and create efficient and maintainable Python applications. Whether you are working on Python web development, software tools, or offering Python services, writing clean and structured code will make a big difference.
Implement these tips in your daily coding routine, and you will see a positive impact on your projects!